02单向循环链表
二、单向循环链表
链表结构体
1 | typedef int DataType; |
1、初始化链表

1 | // 初始化链表 |
2、创建一个节点
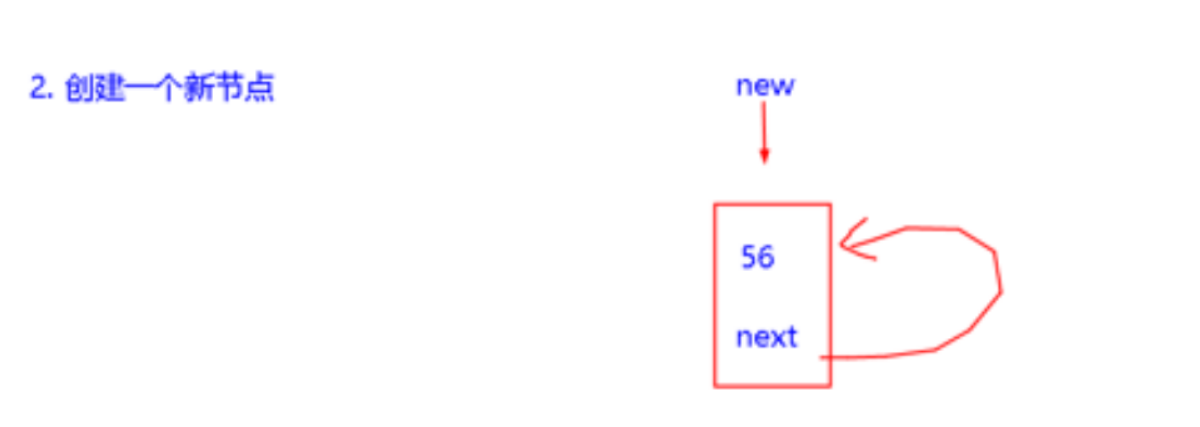
1 | // 创建一个新节点 |
3、尾插
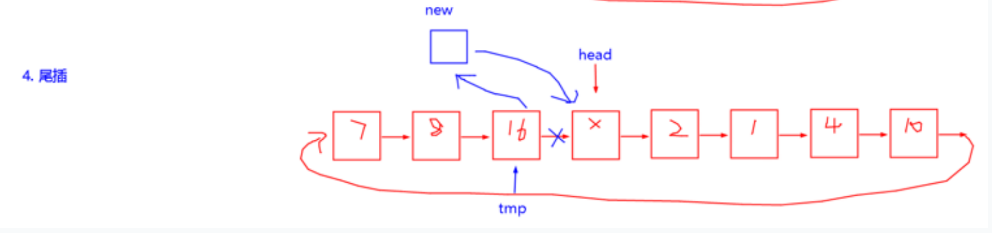
1 | // 在链表的尾部添加一个节点 |
4、头插
1 | // 在链表头部添加一个新节点 |
5、遍历链表
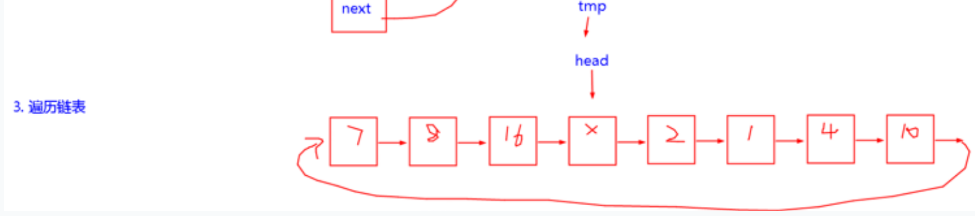
1 | // 遍历函数 |
6、查找节点
1 | // 查找节点 |
7、删除节点
1 | // 删除节点 |
8、更新节点数据
1 | // 更新节点数据 |
9.1、移动节点 (把data所在的节点,移动到n节点之后)
1 | // 移动节点 |
9.2、移动节点 (把data1移动到data2之后)
1 | // 把data1移动到data2之后 |
10、查找节点
1 | // 查找节点 |
11、判断空链表
1 | // 判断空链表 |
12、销毁链表
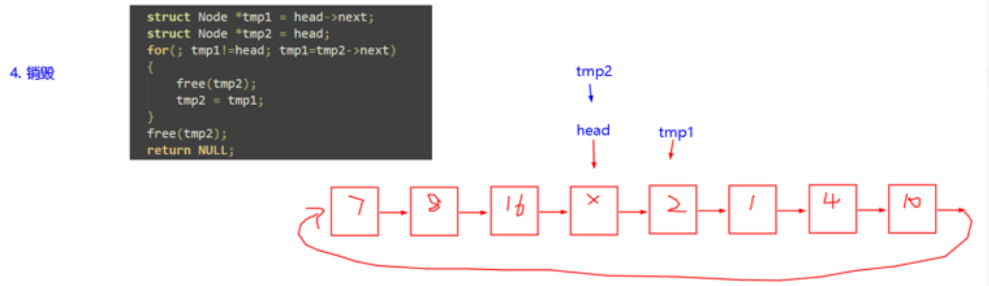
1 | // 销毁链表 |
All articles in this blog are licensed under CC BY-NC-SA 4.0 unless stating additionally.